Mainly there are two types of programming paradigms one being Functional and other is Object-Oriented (OOP). As the names suggests Functional approach emphasizes on pure functions (no state changes and shared data). OOP programming uses classes and objects to model the problem domain.
Lets explain the differences through a small application. Requirement is to create a web application for a company in which users can login, update their Bio. Each user can see other users basic info.

Classes
Class is the blueprint or template of an entity in an application. The class defines the properties (attributes or data) and behaviors (methods or functions).
In the simple application we have a class named Employee. Employee’s name, contact number etc. are the properties and it defines who an employee is. UpdateContact() are the methods and it refers to the actions a employee can do.
Class Examples
Blueprint of a car or a house is a class. These blueprints in itself doesn’t actually do anything unless we create a real car or real house out of the blueprint which we can actually use. Those are objects and interaction of these objects makes the real application which user interacts with.
In an application like Active Directory the user schema is the class and the actual users seen in active directory users and computers console are the objects created out of the user schema.
Objects
Objects are instances of a class. In our example, Steve reports to Joey and below is how their objects will get represented. Both Steve and Joey are objects of type Employee. Each object has it’s own identity in properties and methods like employee name, contact etc. as defined in the Employee class.
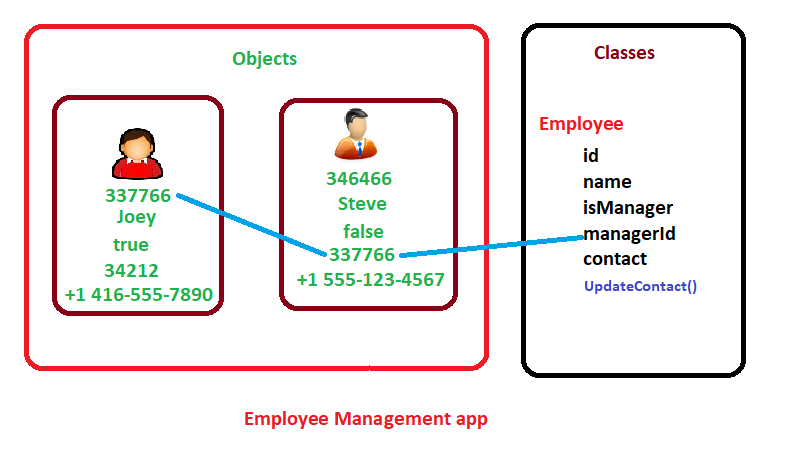
Real World Applications
In real world or large applications are made of lot of classes. Just extending our simple application to include handling of timesheet, computer information for each employee, Alerts on expense reports etc. we can imagine lot more of classes.
Each of these classes creates objects and they interact and compose within and with each other to make real world large application.

Leave a Reply